Maps JavaScript: A concise and simple overview
Do you wonder what maps in JavaScript look like and how you can use them? In this post, we will look at what a map is and how it differentiates from objects. Additionally, we will also learn to create and use them effectively!
What are maps?
Maps are a data structure, and in JavaScript, they are implemented as a class. Additionally, they are not related to the array function map(). If you are interested in learning how that function works, check out this post here.
A map is a list of key-value pairs, where the key can either be an object or any primitive data type (learn more about data types here). The catch is that the map remembers the order in which the entries were inserted.
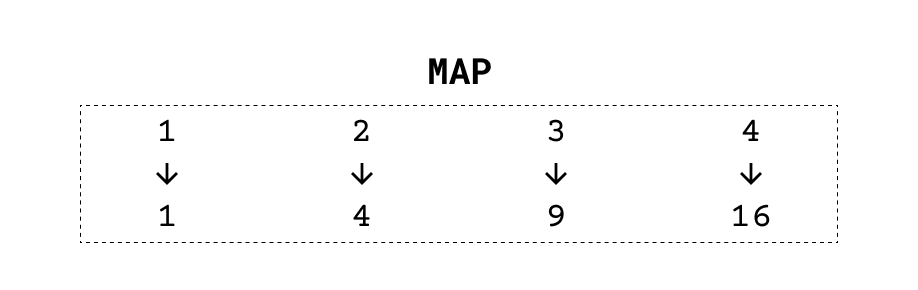
But a map looks pretty similar to a JavaScript object, doesn’t it? Yes, it does, but there are some key differences that we will look at now.
Differences from Maps to JavaScript Objects
It’s true. Both JavaScript objects and maps are pretty similar. You can insert new elements, retrieve them, and remove them. For that reason, objects were used as maps before the introduction of maps.
So, let’s look at the key differences between the two structures. The first key difference is that, as already stated, the map remembers the insertion order of its elements. This can, for example, help when you want to show somewhere the order of the elements you received.
Need help or want to share feedback? Join my discord community!
The second and more important difference is that you can use objects and any primitive data type as a key. For objects, you are only allowed to use strings (or everything that can be converted into a string), and that is very limiting. For example, with maps, you can map objects to other objects, like a person object to an address object!
How to create maps in JavaScript?
Creating maps in JavaScript is straightforward. You can do it by calling the constructor of the map class with the new
keyword. In case you do not understand this, don’t worry. Check out this post explaining the basics of classes here!
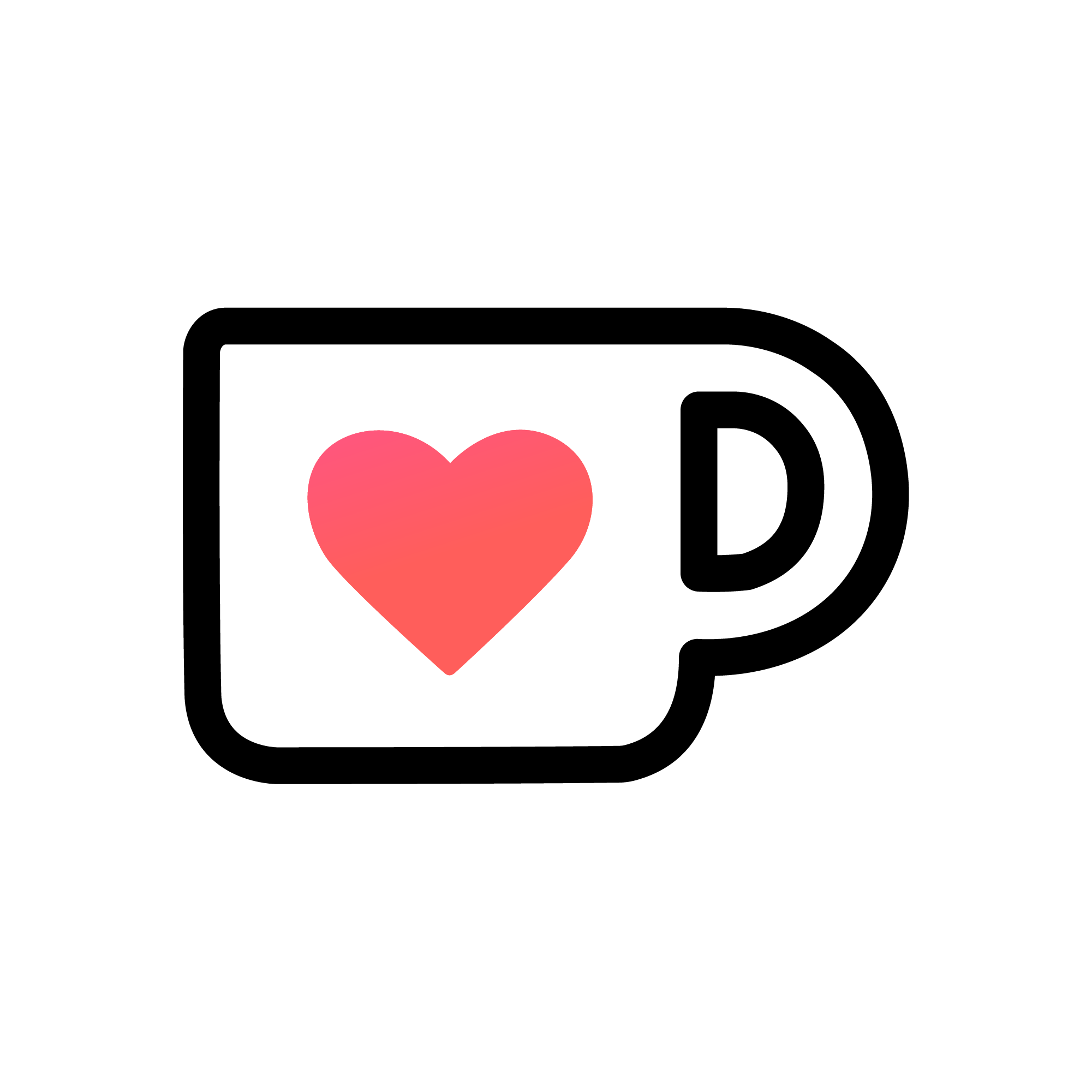
If this guide is helpful to you and you like what I do, please support me with a coffee!
You have multiple options with the constructor. The first one is to call the constructor without any parameter. In this way, you will create an empty map that you can fill with elements at a later point.
let empty = new Map();
The second option is to create the map with some initial values. For that, you have to give the constructor an iterable (e.g., an array) containing the key-value pairs. That would look like this:
let squares = new Map([
[1, 1],
[2, 4],
[3, 9]
]);
With this, we created our first map, where the values are the squares of our keys. In the next section, we will work with this map!
Work with Maps in JavaScript
Now we will look at the most important functions to work with maps! Let us start with the basics of creating, retrieving, and removing data. We will use and modify the squares map created in the previous section for the explanation.
Creating data
First, let’s add the next squared value to the map. For that, the key should be 4, and the value should be 16:
squares.set(4, 16);
Now let’s check if adding the value was successful. For that, we will check if the size is 4:
console.log(squares.size); // 4
Retrieving data
Then let us retrieve the value of key 3. But before doing that, we should check if key 3 exists in the map:
let keyExists = squares.has(3);
if(keyExists)
console.log(squares.get(3)); // 9
Deleting data
Lastly, let’s delete some data from the map. For that, we have two methods. We can either remove one specific key-value pair or clear the whole map. For the first option, you have to use the delete method with the appropriate key. In this example, we want to delete the first entry (key 1) and then check if it was removed successfully:
squares.delete(1);
if (!squares.has(1))
console.log("Key 1 deleted"); // Key 1 deleted
For the second option, you can use the clear function. It removes all available entries from the map:
squares.clear();
if (!squares.has(2) && !squares.has(3) && !squares.has(4))
console.log("All pairs deleted"); // All pairs deleted
Iterating over JavaScript maps
After learning the basic functions, let’s look at the different ways to iterate over JavaScript maps.
There is not only one but multiple ways to iterate over the different components of maps. First, you can use the for…of loop to iterate over keys, values, and elements. That would look like this (for this, we will restore the state of squares before deleting entries):
// keys
for (let key of squares.keys()) {
console.log(key); // 1 | 2 | 3 | 4
}
// values
for (let val of squares.values()) {
console.log(val); // 1 | 4 | 9 | 16
}
// entries
for (let entry of squares.entries()) {
console.log(`${entry[0]}:${entry[1]}`); // 1:1 | 2:4 | 3:9 | 4:16
}
// entries simpler
for (let [key, value] of squares.entries()) {
console.log(`${key}:${value}`); // 1:1 | 2:4 | 3:9 | 4:16
}
In my opinion, the second way of using entries with the for…of loop is really intuitive. It works by using destructuring, a method where you give names to different indexes of an array. In our case, each entry is an array of 2 elements, and with the restructuring, we use “key” for the first and “value” for the second index. To learn more about restructuring, check this post here.
Additionally, you can use a forEach loop with a callback function (to learn more about callback functions, read this post here):
squares.forEach(
(value, key, map) => console.log(`${key}:${value}`) // 1:1 | 2:4 | 3:9 | 4:16
);
Conclusion
In this post, we learned what JavaScript maps are, how to create them, and how to work with them. For that, we had a look at how to create new entries, retrieve entries and delete entries. Additionally, we also got a short overview of the different ways to iterate over maps.
In my opinion, one of the biggest benefits of using maps is that it is the data structure created for maps and that you can use nearly everything as a key.
I hope this post was helpful to you and gave you an overview of maps. If you have any questions or feedback, feel free to leave a comment, I would love to hear from you. Additionally, consider subscribing to my newsletter to get informed about new posts!
Add Comment