Arrow Functions in JavaScript: A simple Introduction
Do you want to learn more about arrow functions in javascript? In this post, we will learn more about anonymous functions as the base for arrow functions, how you create arrow functions and what problems can occur by using them.
What are arrow functions in javascript?
Arrow functions are a new and shorter way to write anonymous functions. Since their introduction with ES6, they have been a big part of working with JavaScript.
One big field they are frequently used in are callback functions. Callback functions are functions that you input into another function. You can read and learn more about their use cases here.
One example for an arrow function could be this function multiplying two numbers and returning the result:
let multiply = (a,b) => a*b;
Don’t worry if you do not understand the syntax yet. We will go into more detail in a later section. But, for now, we will first look at anonymous functions because they are the basis for arrow functions!
What is an anonmyous function?
An anonymous function is a function without an assigned name. That has one big side effect. You cannot call this function by using its name!
Need help or want to share feedback? Join my discord community!
However, you can store it inside a variable and call it by calling the variable. But why would you do that? One answer that I liked is that you can reassign the function at a later point and still call it by the variable name (stackoverflow).
The according example is:
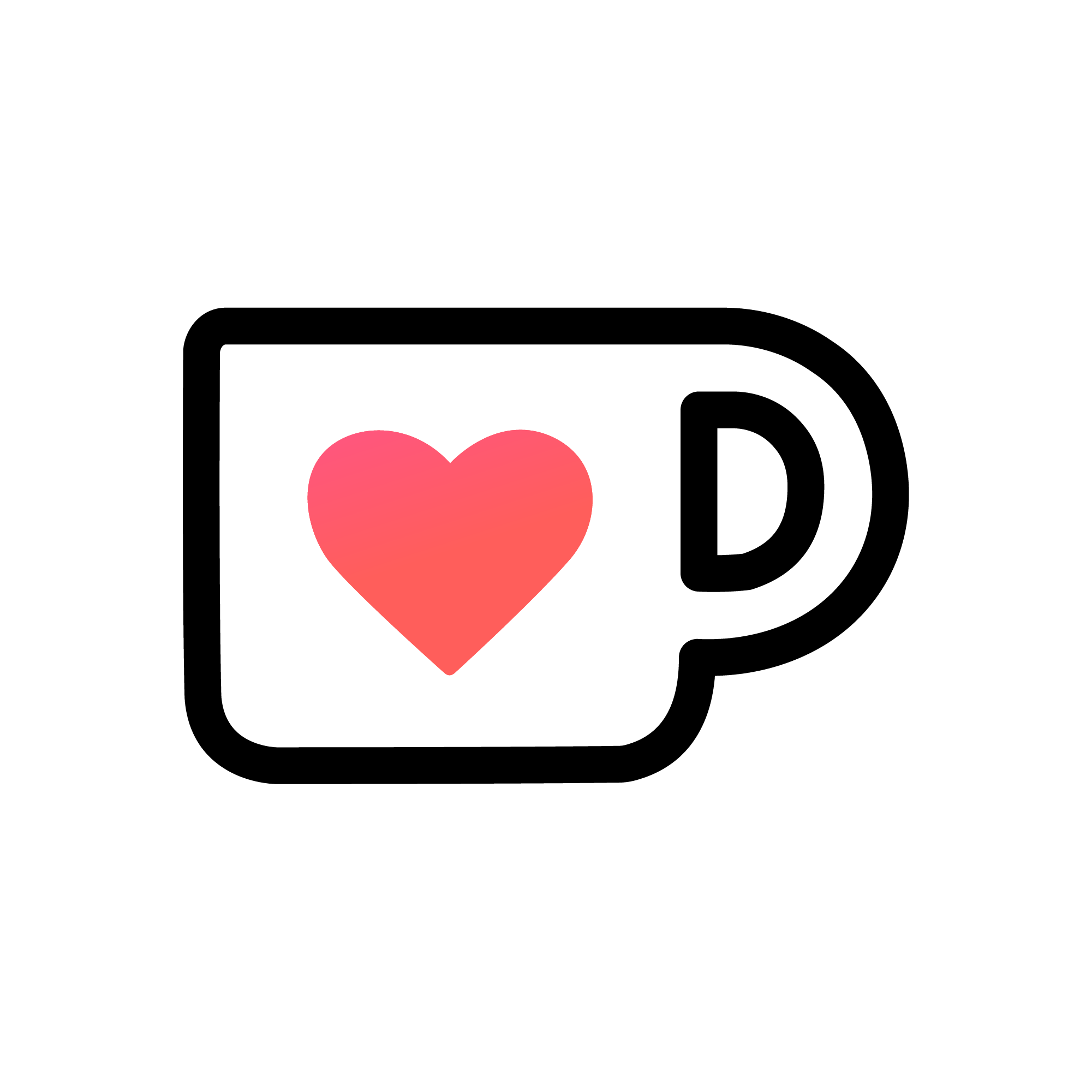
If this guide is helpful to you and you like what I do, please support me with a coffee!
var foo = function(a){ return a * 2; }
var bar = foo(2);
foo = function(a){ return a / 2; }
bar = foo(bar);
From this example, we can also see how to define anonymous functions:
let greeting = function(person) {
return "Greetings" + person;
}
And also how to store it in a variable and call it at a later point.
let greeting = function(person) {
return "Greetings" + person;
}
greeting("Peter");
Arrow functions in detail
As a short recap: Arrow functions are anonymous functions, just written differently. To illustrate that in a better way, we will transform the before-created anonymous function into an arrow function step-by-step. So, in the beginning, the anonymous function looks like this:
let greeting = function(person) {
return "Greetings" + person;
}
To transform the function into an arrow function, we first remove the function keyword and add an arrow between the function contents and the parameter parenthesis.
let greeting = (person) => { return "Greetings" + person; }
This method works for all functions!
In case your anonymous function is a one-liner, as you can see in the example above, you can simplify the syntax even more. You can remove the curly brackets and the return keyword. Removing return is possible because the arrow function without curly brackets returns the value by default. This would transform the function like this:
let greeting = (person) => "Greetings" + person;
Lastly, because this anonymous function has only one parameter (the person), you can also remove the parameter parenthesis like this:
let greeting = person => "Greetings" + person;
The differences between arrow functions and regular functions
In this last section, we will discuss the differences between arrow functions and regular functions in javascript (e.g. anonymous functions, basic functions).
The big difference is the “this” keyword. In a regular function, the keyword represents the object that called the function (e.g. the document, a button, etc.). In an arrow function, the keyword always represents the object that defined the function.
To better understand that, we have the following example:
- One function once as a regular function and once as a arrow function
- The functions are called twice, once while loading the page and once by pressing a button
let reg = function() { console.log(this); };
let arrow = () => { console.log(this); };
//The window object calls the function:
window.addEventListener("load", reg);
window.addEventListener("load", arrow);
//A button object calls the function:
document.getElementById("btn").addEventListener("click", reg);
document.getElementById("btn").addEventListener("click", arrow);
The result is:
- For the regular function “this” represents: window and button
- For the arrow function “this” represents: window and window
For you, the takeaway is that the arrow functions behave differently with the “this” keyword and that you need to use a regular anonymous function if you need the normal behavior.
Conclusion
In this post, we learned everything about arrow functions in javascript, where they come from, how to create them and what problems can occur using them.
As a short recap, an arrow function is an anonymous function written in a more concise syntax.
I hope this post helped you in understanding the arrow function. If that is the case, I would love you to leave a comment and subscribe to my newsletter!
[convertkit form=2303042]