How To Change URL Query Parameters Without Reload (SvelteKit)
Do you want to learn how to change your URL Query Parameters without a page reload in SvelteKit? In this guide, we will learn exactly that by looking at a super quick example.
Change URL Query Parameters without Reload
To change the URL Query Parameters without a reload, we will first create a page-wide variable containing all possible search parameters. When we want to change one of those, we will set the one we want to change and then navigate to a URL containing all of them.
The following code contains an example with two variables and a function that changes both of them.
<script lang="ts">
import { goto } from "$app/navigation";
import { page } from "$app/stores";
const searchParams = new URLSearchParams("docs=false&page=1");
function go() {
searchParams.set("docs", Math.random() > 0.5 ? "true" : "false");
searchParams.set("page", Math.random() > 0.5 ? "1" : "2");
goto(`?${searchParams.toString()}`);
}
$: docs = $page.url.searchParams.get("docs") === "true";
$: site = parseInt($page.url.searchParams.get("page")?.toString() || "0");
</script>
The important parts here are to create the URLSearchParams
object, set a specific parameter, and call goto on all parameters inside the functions. To make the parameters available on the page, we create variables for them.
Applying it to a table
Now that we know how it works in principle, we will apply this knowledge to a practical example: a table containing people’s data. The styling is done using SkeletonUI.
The following component can be copied into its own page to follow along with the tutorial.
<script lang="ts">
import { goto } from "$app/navigation";
import { page } from "$app/stores";
const data = [
{
name: "Felix",
age: 20,
gender: "m"
},
{
name: "John",
age: 30,
gender: "m"
},
{
name: "Jane",
age: 40,
gender: "f"
},
{
name: "Peter",
age: 50,
gender: "m"
}
];
let mutatedData = data;
</script>
<div class="container h-full mx-auto flex justify-center items-center">
<!-- Responsive Container (recommended) -->
<div class="table-container w-80">
<!-- Native Table Element -->
<table class="table table-hover">
<thead>
<tr>
<th class="table-sort-{sorted}"><button on:click={sortName}>Name</button></th>
<th><button on:click={filterAge}>Age</button></th>
<th>Gender</th>
</tr>
</thead>
<tbody>
{#each mutatedData as person}
<tr>
<td>{person.name}</td>
<td>{person.age}</td>
<td>{person.gender}</td>
</tr>
{/each}
</tbody>
</table>
</div>
</div>
As you can see, we still need to create two functions, but before we do so, we will follow the steps outlined above. We will first create a searchParams variable containing the query parameters sort and ageMin. In addition, we will also create the variables containing the query parameters.
Need help or want to share feedback? Join my discord community!
const searchParams = new URLSearchParams("sort=asc&ageMin=0");
let sorted = 'asc';
let greaterThan = 0;
Next, we will create a reactive declaration that updates the mutated data based on the changing query parameters. The two things shown in this guide are to sort the data based on names and filter based on ages.
$: {
sorted = $page.url.searchParams.get("sort") || 'asc';
greaterThan = parseInt($page.url.searchParams.get("ageMin")?.toString() || '0');
if (sorted === 'asc') {
mutatedData = data.sort((a, b) => b.name.localeCompare(a.name));
} else {
mutatedData = data.sort((a, b) => a.name.localeCtyompare(b.name));
}
mutatedData = data.filter(person => person.age > greaterThan);
}
And lastly, we create the two functions to update the query parameters.
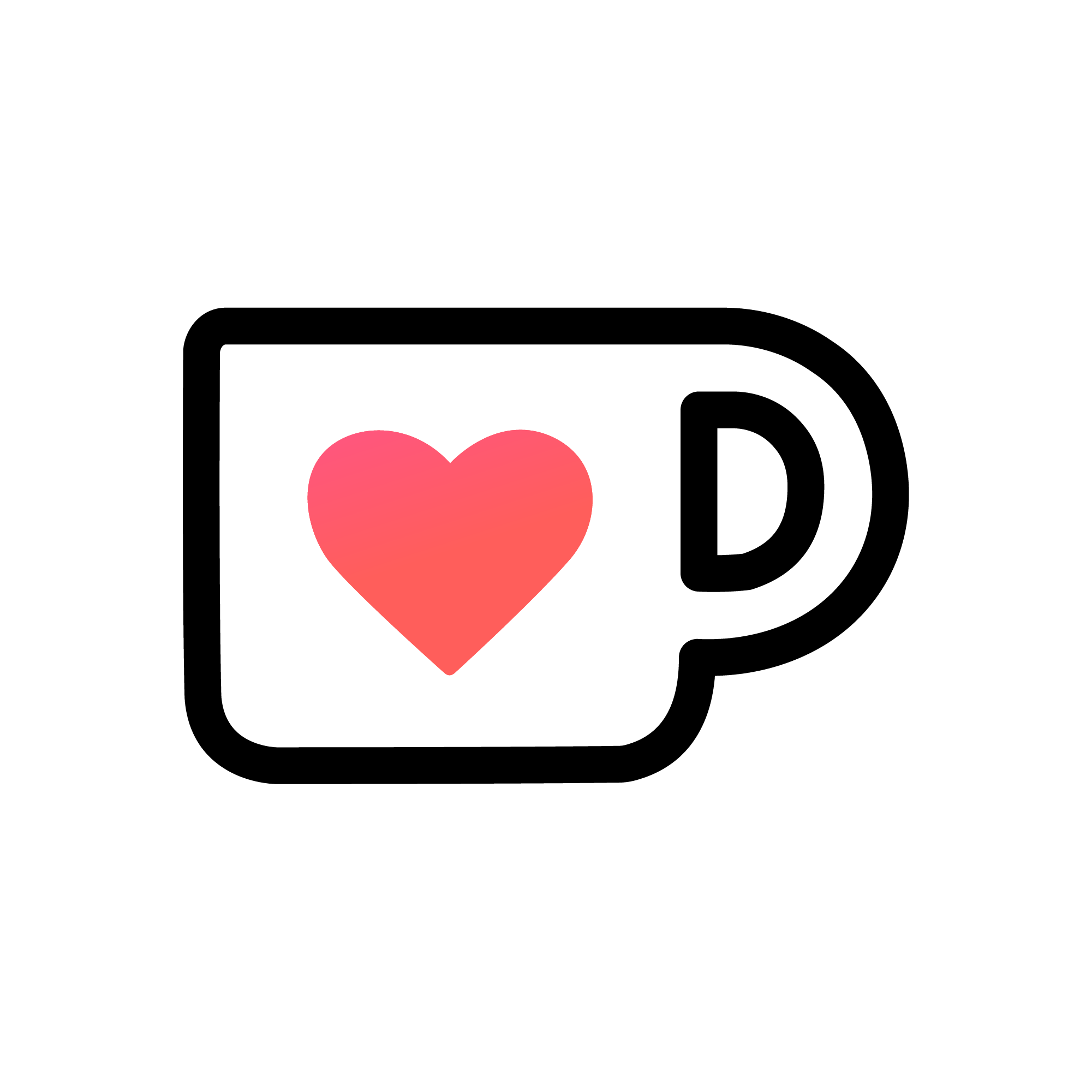
If this guide is helpful to you and you like what I do, please support me with a coffee!
function sortName() {
if (sorted === 'asc') {
searchParams.set("sort", "dsc");
} else {
searchParams.set("sort", "asc");
}
goto(`?${searchParams.toString()}`);
}
function filterAge() {
if (greaterThan === 0) {
searchParams.set('ageMin', '30')
} else {
searchParams.set('ageMin', '0')
}
goto(`?${searchParams.toString()}`);
}
And with that, we have a table with filterable and sortable columns.
Conclusion
In this guide, we learned how to change URL query parameters without a reload in SvelteKit. The main steps are to create a searchParams variable and mutate the parameters in there. To then navigate, you need to call the goto
function by converting the searchParams to a string.
I hope this guide is helpful to you, and if you have any questions, feel free to ask in the comments! And if you want to get updated on my latest posts, feel free to subscribe to my newsletter!
Add Comment