JavaScript Callback Functions: A simple overview with examples
Are you interested in learning about JavaScript callback functions? In this post, we will look at what a callback function is, how you can create it, and in addition to that, we will also check on the differences between synchronous and asynchronous callbacks.
Be excited because callbacks are a cool, useful, and important concept in programming!
What are Callback Functions in JavaScript?
A callback function (B) is a basic function that you input into another function (A). Function (A) then calls the function (B) inside the code. In case you do not know about functions yet, check out this post here. In the next steps, we will create an example to illustrate how function (A) and function (B) work together.
Let’s start by creating two functions, one to display the code inside an HTML tag and one to multiply two numbers. (The goal is always to display the multiplied numbers somewhere!)
// (B)
function display(input) {
document.getElementById("show").innerText = input;
}
// (A)
function multiply(num1, num2) {
let res = num1 * num2;
return res;
}
After creating both functions, we can use them together in two different ways. The first option is to call both functions after each other.
let res = multiply(5, 5);
display(res);
With the following result (this stays the same with all other options, where using the display function):
Need help or want to share feedback? Join my discord community!
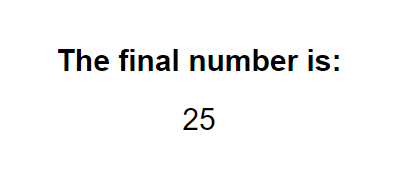
The second option is to call function B inside of function A. For that, we have to change function A like this (we still have the return, so we can also use the value differently):
// (A)
function multiply(num1, num2) {
let res = num1 * num2;
display(res);
return res;
}
multiply(5, 5);
The result for both options is the same. We multiply 5 and 5 and then display 25 inside of our HTML. The first option, in this case, is more versatile because we also have the possibility to print the result into the console without having to change the multiply code. On the other hand, we always want to display the multiplied numbers somewhere, so it does not make sense to always call the two functions separately. So, what should we do?
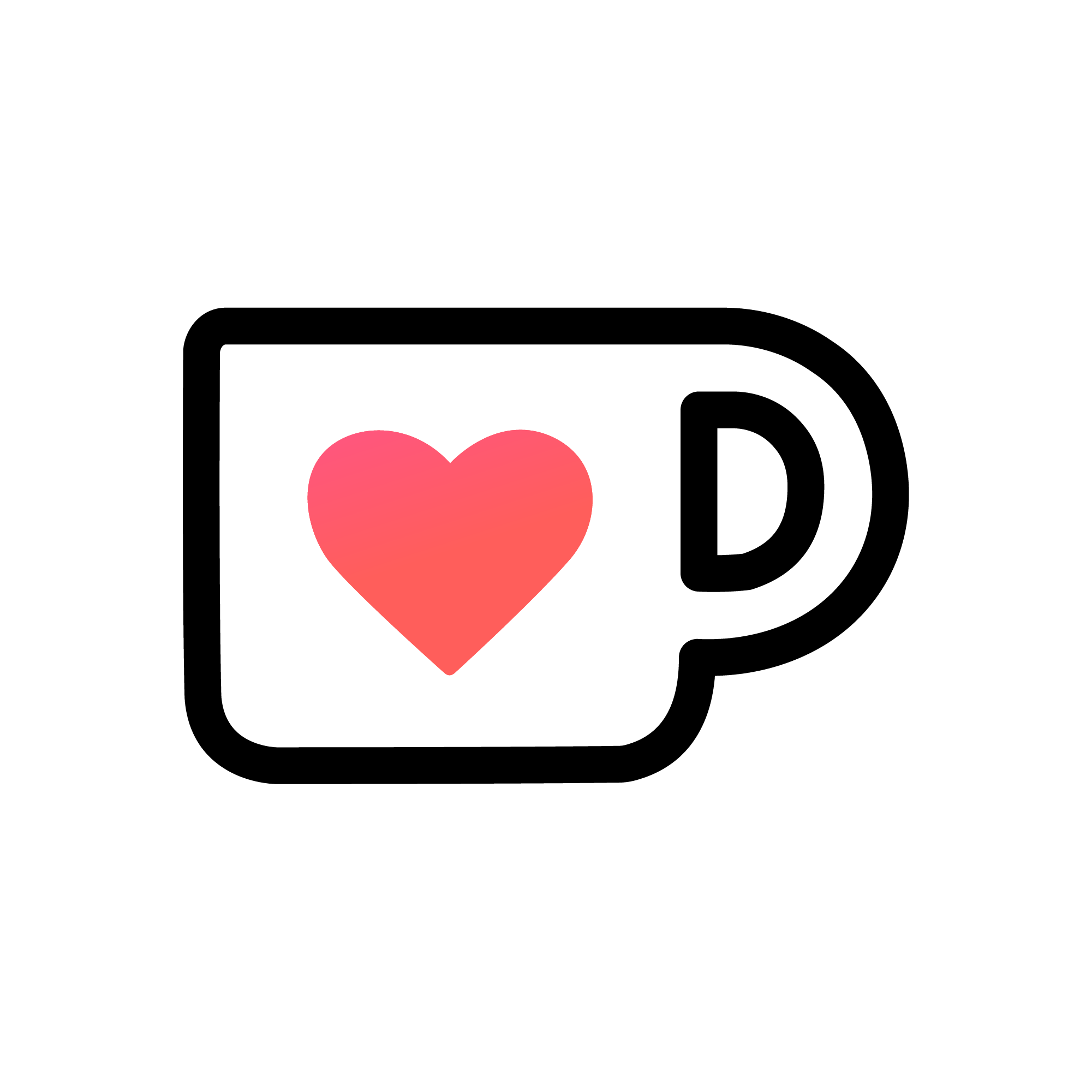
If this guide is helpful to you and you like what I do, please support me with a coffee!
Basic callback
For exactly this case, we can perfectly use callback functions. But, first, we have to change function (A) to take a function as an input parameter. And then, inside of function (A), the input function is called:
// (A)
function multiply(num1, num2, cb) {
let res = num1 * num2;
cb(res);
return res;
}
That way, we can hand over our function (B) to function (A) to display the result inside of the HTML, like this:
multiply(5, 5, display);
Important: Here, we just input the name of the function. We do not explicitly call the function with parenthesis.
The result is as expected, and we see the number 25 displayed inside our HTML.
One of the benefits of a callback function is that I can input whatever function I like. So, for example, I can change the function I hand over from display to console.log and write the number 25 into the console instead of the HTML:
multiply(5, 5, console.log);
Anonymous callback
Another cool thing we can do is use anonymous functions to input a custom function into the method. To learn more about anonymous or arrow functions, check out this post here.
In the following example, I will create an anonymous function to display and log the result simultaneously:
multiply(5, 5, (res) => {
console.log(res);
display(res);
});
Empty callback
Lastly, we can also do nothing with the result without any problem and, for example, use the number 25 for something completely different:
let useless = multiply(5,5);
Synchronous callback functions
Synchronous means that the instructions are called one by one and one after the other. You saw this already in the example above. The function will execute one by one. The only difference is that you can input functions into it and change what the function does with the data.
Some examples of synchronous callbacks are the array.forEach function or similar. You call the function on the array and then define what happens with each element in the array:
array.forEach((element) => {
console.log("The element is:", element);
});
Asynchronous callback functions
Asynchronous means that the instructions are called one after the other, but they are not executed in the same order. The asynchronous callback executes after the higher-order function (the one that calls the callback) finishes or on a specified event because they are non-blocking.
One example is the setTimeout function that calls the defined callback function after a certain time. All other functions afterward still execute because asynchronous functions and callbacks are non-blocking.
console.log(1);
setTimeout(() => console.log(2), 2000);
console.log(3);
As described before, the print order will be: 1 3 2, because the console.log(2) executes after 2 seconds.
You can also create an asynchronous callback yourself by utilizing async and await. However, asynchronous programming is a completely different thing. For a basic understanding, you can check out this post here. In the following example, we will create an async callback. Our callback will retrieve a fact about the first number while the function calculates the multiplication result:
function multiply(num1, num2, cb) {
cb(num1);
return num1 * num2;
}
multiply(5, 5, async (input) => {
let fact = await fetch("<http://numbersapi.com/>" + input + "/math?json=true");
let res = (await fact.json()).text;
console.log(res);
});
Conclusion
In this post, we learned about javascript callback functions and their existence in different spheres. The basic callback in a synchronous environment and then the asynchronous callback functions you can create yourself with async and await.
I hope this post was helpful for you, and you got a basic understanding of callbacks. In case you are interested in more content like that, consider subscribing to my newsletter!
Add Comment