JavaScript Arrays: Learn everything you need to know
JavaScript Arrays are used in a variety of different programs! Therefore, mastering them will make you a better developer and let you achieve more in less time! Consequently, we will look at how to modify the elements inside the array and how to work with the array functions to save you a lot of time and effort!
What are JavaScript Arrays
Arrays are list-like objects, and they can store multiple values. You can access each of the values individually with a given index. The index of the first element is 0, the index of the second element is 1, and so on.
Important to note is that arrays do not have a specific type nor a specific length. Resulting from that, you can store as many values in there as you want (max. is around 4.29 billion elements according to this answer here), and you can also remove and add new elements at a later point.
Additionally, arrays provide multiple methods that ease working with them a lot. One example is the filter method, which allows you to remove all elements that do not meet a certain condition.
Create JavaScript Arrays
Creating JavaScript arrays is a straightforward process. You can create them by assigning a list of values inside of square brackets to a new variable like this:
let fruits = ["Apple", "Banana", "Orange"];
The following example visualizes the array and the index of each element:
Need help or want to share feedback? Join my discord community!
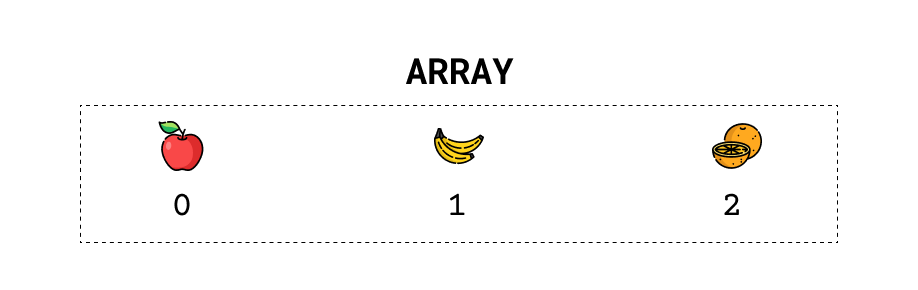
Next, we will look at manipulating the elements inside the array. First, we will learn how to add a new element and how to access and update them. After that, we will learn how to remove elements from the array, and lastly, we will learn about some of the other functions provided by arrays.
Work with JavaScript arrays
To explain how you can work with JavaScript arrays, we will use the previously created fruits
array. A possible basic thing you want to do with arrays is to modify and access the elements inside.
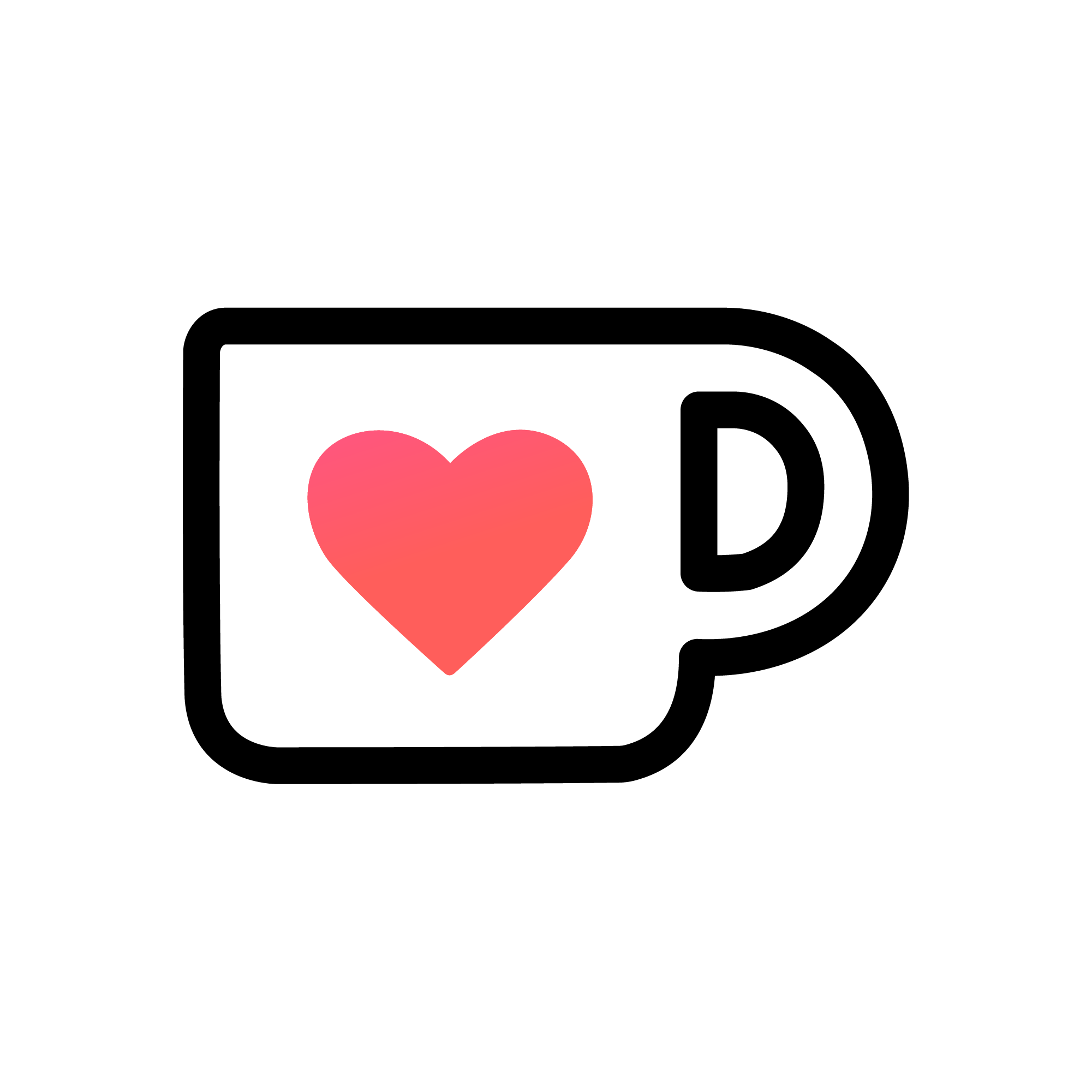
If this guide is helpful to you and you like what I do, please support me with a coffee!
Add Elements
Adding elements to JavaScript arrays can be achieved in two ways. The first is to add a new element at the end of the array. You can do that by either using the current length of the array like this:
fruits[fruits.length] = "Mango";
Or in a safer and the recommended way with the push method like this:
fruits.push("Mango");
The other way is to add a new element at the beginning of the array. To do this, you have to use the unshift method like this:
fruits.unshift("Lemon");
Access Elements
You can access array elements by using their index. Our array currently looks like this, and the elements have indexes from 0 (Lemon) to 4 (Mango). In the following example, we will access the first and last elements of the array.
For the first element, we have to use the index 0, and for the last element, we have to use the index 4. That number results from the array’s length minus one (in programming, you mostly start counting from 0). So we can also use this equation to access the last element:
// access the first element
let first = fruits[0]
// access the last element
let last = fruits[fruits.length - 1]
console.log(first, last); // Lemon Mango
Update Elements
To update an element, we first have to access it with the index and then assign a new value. In the following example, we change the banana to a dragon fruit:
fruits[1] = "Dragon Fruit";
Remove Elements
The recommended way to remove elements from an array is to use the methods shift and pop. shift removes the first element of an array, whereas pop removes the last element of an array. We can see that in the following example good:
// remove first and last element
fruits.shift()
fruits.pop()
console.log(fruits); // ['Dragon Fruit', 'Banana', 'Orange']
But what if you want to remove a specific element in the middle of an array? For that, you can use the delete keyword. The problem with this is that it will leave the element undefined and not move the other elements in its position. Sometimes you want this behavior; sometimes, you don’t. For the cases where you do not want this, you could use the following approach:
// get index of element to delete (you can also just give the index of 1)
delete fruits[fruits.indexOf('Banana')]
console.log(fruits) // ['Dragon Fruit', empty, 'Orange']
fruits = fruits.filter(el => el !== undefined);
console.log(fruits) // ['Dragon Fruit', 'Orange']
With filter, we can remove all the elements changed to undefined after using the delete keyword. We will look at the filter function closer in the next section.
Iterating JavaScript Arrays with Array Functions
For the following functions, we reset the state of our fruits array to this:
fruits = ['Lemon', 'Dragon Fruit', 'Banana', 'Orange', 'Mango']
All of the following functions (excluding indexOf) take callback functions as a parameter. A callback function is basically a standard function that you hand over to another function. To read more, check out this post here.
Additionally, the functions do not change the existing array. To update the current array, you must assign the return value to the same variable again. An example of this is visible in the delete part of the last section.
forEach
The forEach function loops over each element and executes the callback function with the element as input. In the following example, we use the forEach to print out each element individually:
fruits.forEach(el => console.log(el)); // Lemon | Dragon Fruit | Banana | Orange | Mango
map
On the other hand, the map lets you transform your array from one state into another. So, for example, we could change every element to be an Orange because Oranges are great!!!
let oranges = fruits.map(el => "Orange");
console.log(oranges) // ['Orange', 'Orange', 'Orange', 'Orange', 'Orange']
filter
The filter method enables you to remove unwanted elements from your array. For example, in case we only want fruits that have less than six letters, we can do this by checking if the word length is less than six (with the callback):
let shorts = fruits.filter(el => el.length < 6);
console.log(shorts) // ['Lemon', 'Mango']
reduce / reduceRight
With reduce and reduceRight, we can combine all of our elements into a single component. The difference between reduce and reduceRight is that reduce starts from the left side (start) and reduceRight from the array’s right side (end). So, we could combine all fruits into one string, for our example.
let left = fruits.reduce((total, value) => total + ", " + value);
console.log(`I love the following fruits: ${left}`);
// I love the following fruits: Lemon, Dragon Fruit, Banana, Orange, Mango
let right = fruits.reduceRight((total, value) => total + ", " + value);
console.log(`I love the following fruits: ${right}`);
// I love the following fruits: Mango, Orange, Banana, Dragon Fruit, Lemon
every
The every function can be used to check if all elements fulfill a certain condition. For example, we could check if all fruits have less than six characters. For this, we will first check our current array, which should return false, and the array we modified with filter, that should return true:
let valid = fruits.every(el => el.length < 6);
console.log(valid); // false
let shortsValid = shorts.every(el => el.length < 6);
console.log(shortsValid); // true
some
And with some, we then can check if there are at least some elements with less than 6 in the fruits array (as we know, because we did not get an empty array after filtering):
valid = fruits.some(el => el.length < 6);
console.log(valid); // true
indexOf
And lastly, the function indexOf, which we already used in the section above. With this function, we can retrieve the index of a certain element in the array. And we can use this, for example, to delete or update an element with a certain value like this:
let oidx = fruits.indexOf("Orange");
fruits[oidx] = "Super Orange";
console.log(fruits); // ['Lemon', 'Dragon Fruit', 'Banana', 'Super Orange', 'Mango']
As you can see, having these array functions in mind can save you a lot of time, and I would recommend you to try and implement them as often as you can.
Get an overview of all functions here.
Conclusion
Arrays are a main component of JavaScript. They enable you to store different types of data and work with this data intuitively. You can add, update, remove, and of course, access the various elements in an array as you like. Additionally, the array functions enable you to get results in a super-fast way. For example, I use the filter function a lot in projects because it is intuitive and eases working with JavaScript Arrays.
I hope this post was a helpful introduction to JavaScript Arrays and that you now know how to use them!
In case you liked this post, consider subscribing to my newsletter!
[convertkit form=2303042]