JavaScript Dates: A useful guide to date objects
Are you interested in JavaScript Dates, how you can use them, and how you can access different date formatting options? Then you are in the right place. In this post, we will look into all these topics.
We will start exploring JavaScript date objects by learning how to create, update, and access them.
- JavaScript Dates
- Date Objects in JavaScript
- Get Date in JavaScript
- Set Date in JavaScript
- Date Formatting JavaScript
- Conclusion
JavaScript Dates
You will encounter JavaScript Dates in many different applications. They are used to track the date and the time. So as soon as you want to display some time-related stuff or need to work with time in your logic, you have to deal with them.
Some examples where you need the JavaScript Dates are:
- Display the current time in your application
- Display a graph whose values change over time
- Create log messages that include time and date for better tracking (to learn how to create helpful log messages check this post here)
Date Objects in JavaScript
As we learned in the last section, the date object does contain not only a date but also a time! In the following visualization, you can see all components the date object consists of:

Each of these components is accessible and can be used in various ways. For example, if you want to display the time but do not show the seconds, you can access the hour and the minutes!
Need help or want to share feedback? Join my discord community!
After learning this, let’s create our first date object! We have four different ways to do that and they are all displayed in the following code snippet:
new Date()
new Date(year, month, day, hours, minutes, seconds, milliseconds)
new Date(milliseconds)
new Date(date string)
And now with some more detail. A new date without any input parameters creates a new date object with the current date and time:
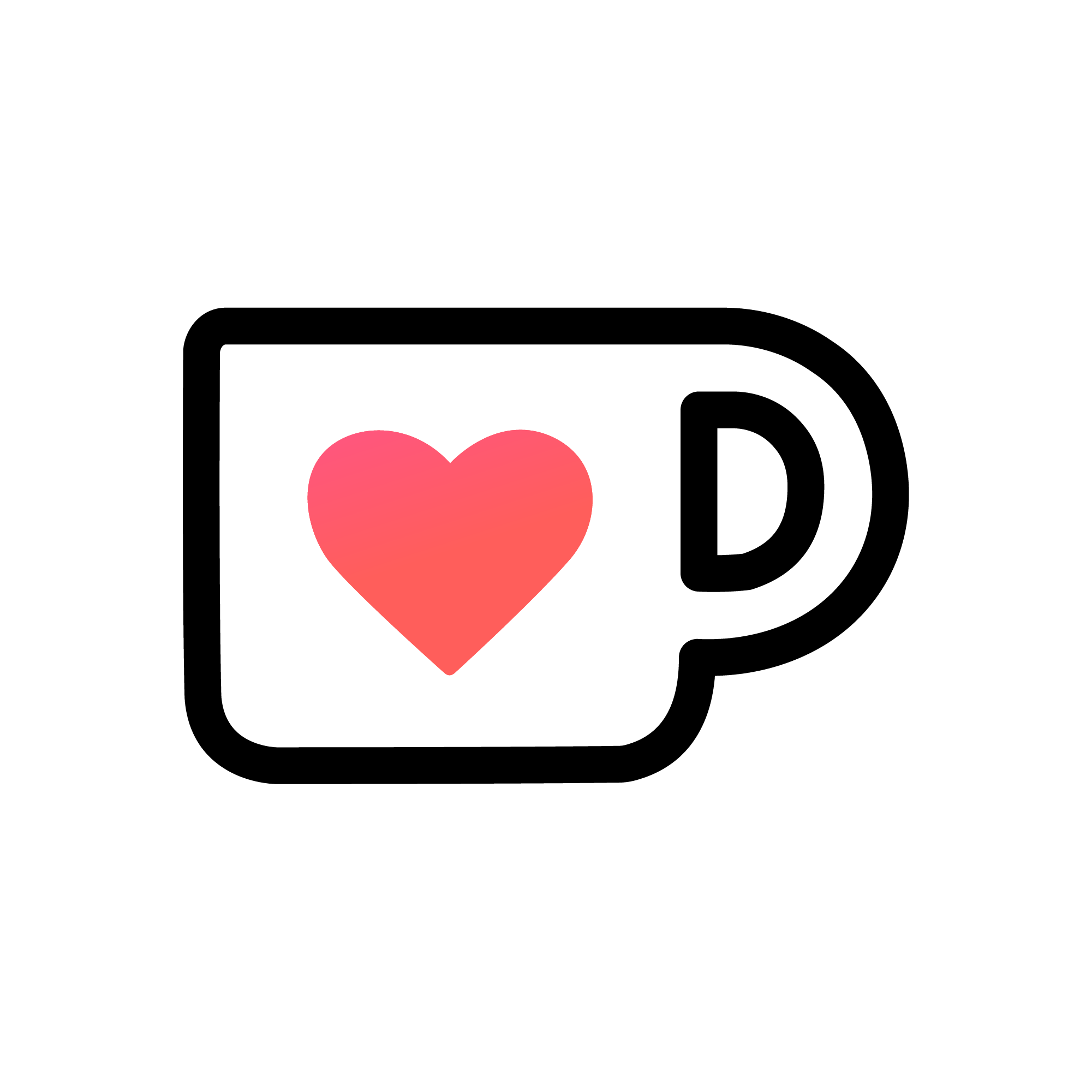
If this guide is helpful to you and you like what I do, please support me with a coffee!
let d = new Date(); //Mon Dec 27 2021 10:17:08 GMT+0100 (Central European Standard Time)
The second way is to give the date object all the information it needs, by specifying the values for each component individually:
let d = new Date(2021, 11, 27, 10, 17, 8, 0); //Mon Dec 27 2021 10:17:08 GMT+0100 (Central European Standard Time)
Important: months are counted from 0 to 11, and in case you input a higher number than that, the difference will be added on top. For example, year=2021 and month=12 will result in January of 2022.
The third option is to input the milliseconds for the specific date. Milliseconds since 1970 (Unix time) is a very popular time format that you will encounter in many different applications. One benefit of working only with milliseconds is that you do not have to care about the various time zones because milliseconds are the same everywhere. You can read more about it here.
let d = new Date(1640596628000); //Mon Dec 27 2021 10:17:08 GMT+0100 (Central European Standard Time)
And lastly, we can also use a date string to create a new date object.
let d = new Date("December 27, 2021 10:17:08"); //Mon Dec 27 2021 10:17:08 GMT+0100 (Central European Standard Time)
We will go more into detail about date strings in the section date formatting JavaScript.
Get Date in JavaScript
You can access different aspects of the date with the date objects getter methods. You got two methods for each property, one to get the information of the date and one to get the same information as the UTC variant. “UTC is the primary time standard by which the world regulates clocks and time”, you can read more about it here.
The methods are listed here, and are named well to know what data you will receive:
Method | UTC Method | Description |
---|---|---|
getFullYear() | getUTCFullYear() | Get the year as a four digit number (yyyy) |
getMonth() | getUTCMonth() | Get the month (0-11) |
getDate() | getUTCDate() | Get the day of the month (1-31) |
getDay() | getUTCDay() | Get the day of the week (0-6) |
getHours() | getUTCHours() | Get the hour (0-23) |
getMinutes() | getUTCMinutes() | Get the minute (0-59) |
getSeconds() | getUTCSeconds() | Get the second (0-59) |
getMilliseconds() | getUTCMilliseconds() | Get the millisecond (0-999) |
getTime() | Get the milliseconds since January 1, 1970 |
For example, you can use the methods to show the current time with hours and minutes, in the console, like this:
let date = new Date();
console.log(`Current time: ${date.getHours()}:${date.getMinutes()}`);
Resulting in this output:

Set Date in JavaScript
As for the getter methods, each property also has one setter method. Setter methods are used to overwrite the current content of a property. In the following table, you can see the methods:
Method | Description |
---|---|
setFullYear() | Set the year (optionally month and day) |
setMonth() | Set the month (0-11) |
setDate() | Set the day of the month (1-31) |
setHours() | Set the hour (0-23) |
setMinutes() | Set the minute (0-59) |
setSeconds() | Set the seconds (0-59) |
setMilliseconds() | Set the millisecond (0-999) |
setTime() | Set the milliseconds since January 1, 1970 |
For example, we update the current day to fit an important appointment:
let appointmentDate = new Date();
appointmentDate.setFullYear(2022,5,1);
console.log(`Appointment at: ${appointmentDate.toDateString()}`);
Results in this output:

We will look at the toDateString method in the next section.
Date Formatting JavaScript
Lastly, we will have a look at date formatting in JavaScript. How to get the date in a specific format, and what strings can be used to create date objects.
There are three main formats, whereby the ISO-Format is the preferred one. ISO is a standard and thus is supported everywhere.
Type | Example |
---|---|
ISO Date | “2015-03-25” |
Short Date | “03/25/2015” |
Long Date | “Mar 25 2015” or “25 Mar 2015” |
The ISO format in total length looks like this:
YYYY-MM-DDThh:mm:ssZ
This means that you can create a Date object with an ISO string consisting of:
Code | Meaning |
---|---|
YYYY | Year |
MM | Month |
DD | Day |
hh | Hour |
mm | Minute |
ss | Second |
So let’s do that:
let d = new Date("2021-12-27T10:17:08Z"); //Mon Dec 27 2021 10:17:08 GMT+0100 (Central European Standard Time)
One more important thing. The Z at the end of the string means that the time is set to UTC, but if we want to modify the time relative to UTC, we can change the Z to +hh:mm or -hh:mm to add or remove the set time.
Per default, printing the object itself will result in this string (created by the toString() method):
Mon Dec 27 2021 10:17:08 GMT+0100 (Central European Standard Time)
In case you want to print them in another format, the date object got multiple methods for that:
Method | Call | Output |
---|---|---|
toGMTString() | d.toGMTString() | Mon, 27 Dec 2021 10:17:08 GMT |
toUTCString() | d.toUTCString() | Mon, 27 Dec 2021 10:17:08 GMT |
toISOString() | d.toISOString() | 2021-12-27T10:17:08.000Z |
toString() | d.toString() | Mon Dec 27 2021 11:17:08 GMT+0100 (Central European Standard Time) |
toDateString() | d.toDateString() | Mon Dec 27 2021 |
toTimeString() | d.toTimeString() | 11:17:08 GMT+0100 (Central European Standard Time) |
toLocaleString() | d.toLocaleString(‘de-DE’, { timeZone: ‘UTC’ }) | 27.12.2021, 10:17:08 |
toLocaleDateString() | d.toLocaleDateString(‘de-DE’, { timeZone: ‘UTC’ }) | 27.12.2021 |
toLocaleTimeString() | d.toLocaleTimeString(‘de-DE’, { timeZone: ‘UTC’ }) | 10:17:08 |
Conclusion
In the post, we learned a lot about JavsScript dates. We now know how we can create them, access the held data, and also how to update dates when needed. Additionally, we learned how to get the date in popular formats like UTC or ISO.
I hope this post was helpful to you, and you are now able to work confidently with JavaScript dates.
In case you are interested in more software- and web-development related stuff, consider subscribing to my newsletter never to miss a new post.
[convertkit form=2634876]