Remove Files From a File Input using Svelte
Do you want to create a File Input with Svelte and allow your users to remove individual files from it? In this guide, we will do exactly that. We will first have a look at the problem and the final outcome, and then we will develop the solution.
Introduction
When using the native file input component in Svelte, we do not have the possibility to remove images easily. We can access them using the bind:files
declarative, but it is read-only, so we cannot simply remove a specific element from the FileList.
In this guide, we will now visualize all elements of the file input in a list, and each element gets an action that removes the element from the list. The final solution will look like this:
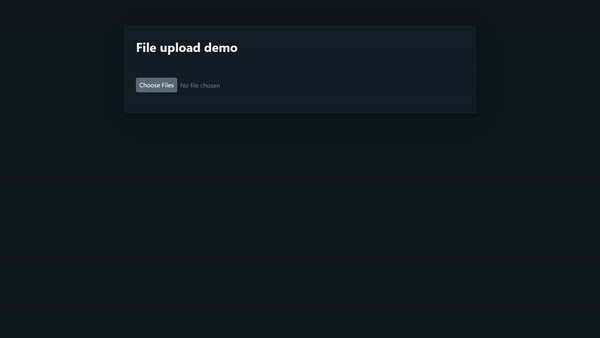
In the next step, we will develop exactly that. The styling of the application is done using Pico.css. And you can find the GitHub Repository here.
Remove files from a file input using Svelte
First, we will create a new svelte component called FileUpload. You can use this component inside other Svelte files using plain Svelte or SvelteKit. The component looks like this:
<input type="file" multiple />
We will now add a script tag and bind the files to a variable. Additionally, we will create a function called removeFile(file)
that creates a new data stream and only adds the files that should not be removed. In the end, it replaces the files
value and thus removes the deleted file from the list.
<script lang="ts">
let files: FileList;
function removeFile(fileToRemove: File) {
const dt = new DataTransfer();
if (files) {
for (let i = 0; i < files.length; i++) {
const file = files[i];
if (file.name !== fileToRemove.name) dt.items.add(file);
}
}
files = dt.files;
}
$: console.log(files);
</script>
<input type="file" multiple bind:files />
{#if files}
{#each Array.from(files) as file}
<div>
<button class="contrast outline" on:click={() => removeFile(file)}>❌</button>
{file.name}
</div>
{/each}
{/if}
<style>
button {
width: max-content;
display: inline-block;
}
</style>
With that, we are now able to remove individual files from the file input in Svelte.
Need help or want to share feedback? Join my discord community!
Conclusion
With that, we created a file upload component that allows us to remove specific files from the native file input. I hope this guide was helpful to you. In case you have any questions, feel free to ask!
Don’t miss out on any updates or future guides by subscribing to my monthly newsletter.
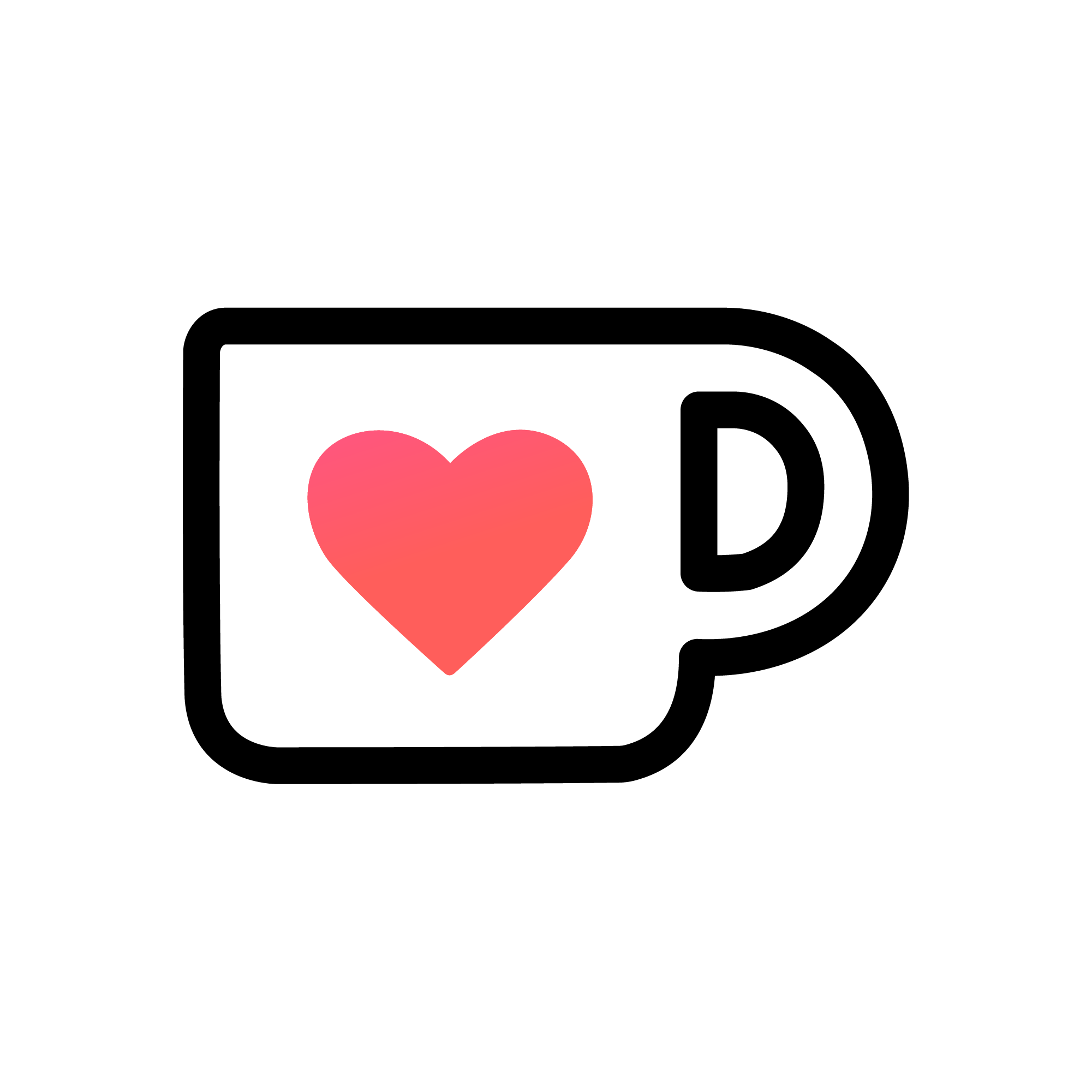
If this guide is helpful to you and you like what I do, please support me with a coffee!
[convertkit form=2303042]