JavaScript Prototypes: Modify existing objects to be helpful for you!
JavaScript Prototypes let you modify object base instances and thus give you the ability to already existing objects. In the following post, we will have look at what a prototype is (explained with a class example) and how to use it. Additionally, we will also look at a practical example that I encountered in one of my projects!
What are JavaScript prototypes
JavaScript Prototypes represent the building structure of an object. So every object inherits its properties and methods from a prototype. An example is a Date object that inherits all its properties from the Date.prototype. Additionally, it works the same for objects that we created.
In my last post, where learned how to use JavaScript classes to create Objects (you can check it out here). So let’s use classes to understand prototypes better. That works well because classes are a good representation of the actual prototype. So to continue, let’s first create a dog class to work with:
class Dog {
constructor(name, age) {
this.name = name;
this.age = age;
}
walk() {}
}
After the creation, let’s have a look at the prototype of the dog:
console.log(Dog.prototype);
/* console
{constructor: ƒ, walk: ƒ}
constructor: class Dog
walk: ƒ walk()
*/
As we can see, the prototype has both the constructor and the walk method.
How to use JavaScript prototypes?
One thing that is important to know is that we cannot add a method to every object instance (here barking) like that:
Need help or want to share feedback? Join my discord community!
Dog.bark = () => console.log('bark');
let bolt = new Dog("Bolt", 3);
bolt.bark();
/* console
Uncaught TypeError: bolt.bark is not a function
*/
This attempt would work if you would execute it on an actual object instance like this:
let tim = new Dog("Tim", 10);
tim.bark = () => console.log('loud bark');
tim.bark();
/* console
loud bark
*/
To add the method to every object instance (already created and new ones) we have to use the prototype property like this:
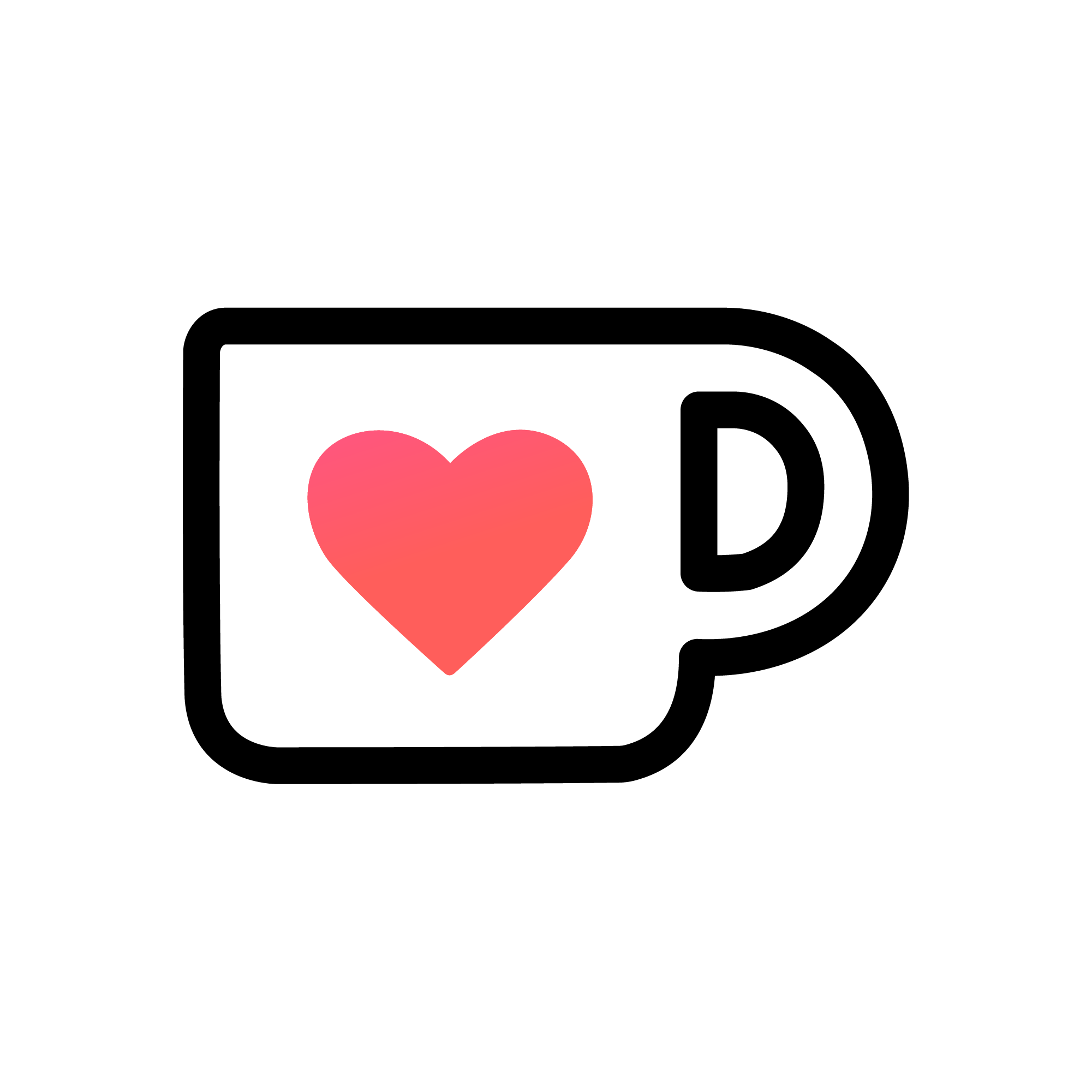
If this guide is helpful to you and you like what I do, please support me with a coffee!
Dog.prototype.bark = function() {
console.log(this.name, "barks");
}
let ben = new Dog("Ben", 6);
ben.bark();
bolt.bark();
/* console
Ben barks
Bolt barks
*/
As we can see, now the function is attached to the instance and can also access the class properties! But be careful when adding new methods with the wish to access class properties because if you want to access them, you have to use function() {}
instead of an arrow function. I explained the reason for how this
works in this post here, so check it out if you are interested.
We can add new methods to a prototype. It also works for properties, although they are not visible in the before shown prototype object. For example, you would add it similar to a method like this:
Dog.prototype.species = "Golden Retriever";
Now all of our dogs are Golden Retrievers, for example, Ben, the dog we created earlier!
These are the basics of JavaScript prototypes. In case you have questions or need help, drop a comment!
Now I will show you one example that made me understand prototypes.
Using prototypes to your advantage (an example)
Okay, up till now, you probably only heard about the idea behind prototypes and not where they can help you. For me, that was the same, and it just made click for me when I used them for the first time!
Now I will show you the example that helped me understand! So, I am currently creating a small Websocket application without using the well-known Socket.io library. Instead, I am using a bit lower-level library ws. In the application itself, I want to send messages to the client, and all of these messages are supposed to be JSON’s. Normally, I would have to do it like this every time:
ws.send(JSON.stringify(
{
type: 'code',
params: {
code: id
}
}));
But with prototypes, I was able to add a new method to the WebSocket. This method now always automatically stringifies my JSON (convert the JSON to a string), and I only have to input the object. It looks like this:
// use function because of this
WebSocket.prototype.sendJSON = function (msg) {
this.send(JSON.stringify(msg));
}
And I now can send messages like this:
ws.sendJSON({
type: 'code',
params: {
code: id
}
});
That now saves me a little bit of typing work and helped me to understand prototypes. So I suggest you look for opportunities to try them in your projects, which will help you figure them out!
Conclusion
In this post, we learned what JavaScript prototypes are and how we can use them to our advantage! In short, we can add a function or property to a prototype like this (if we want to access properties of the prototype we have to use an anonymous function instead of an arrow function, to learn why read here):
obj.prototype.property = value;
obj.prototype.method = function() {console.log(this.property)}
I hope this post helped you in understanding JavaScript prototypes! If you have any questions feel free to ask and if you liked this post consider subscribing to my newsletter!
[convertkit form=2303042]