How To Build A Chrome Extension (Step-By-Step With Example)
Do you want to build your very own Chrome Extension? I was curious too, and in this guide, I will share with you how to build a Calculator extension step-by-step! We will first set up the project, and from there, you can either build your own extension or follow along by building a simple calculator.
- Set up a project to build a Chrome Extension
- Build the calculator as a popup Chrome extension
- Use Chromes storage API to persist the equation and result
- Conclusion
The following GIF shows the final extension:
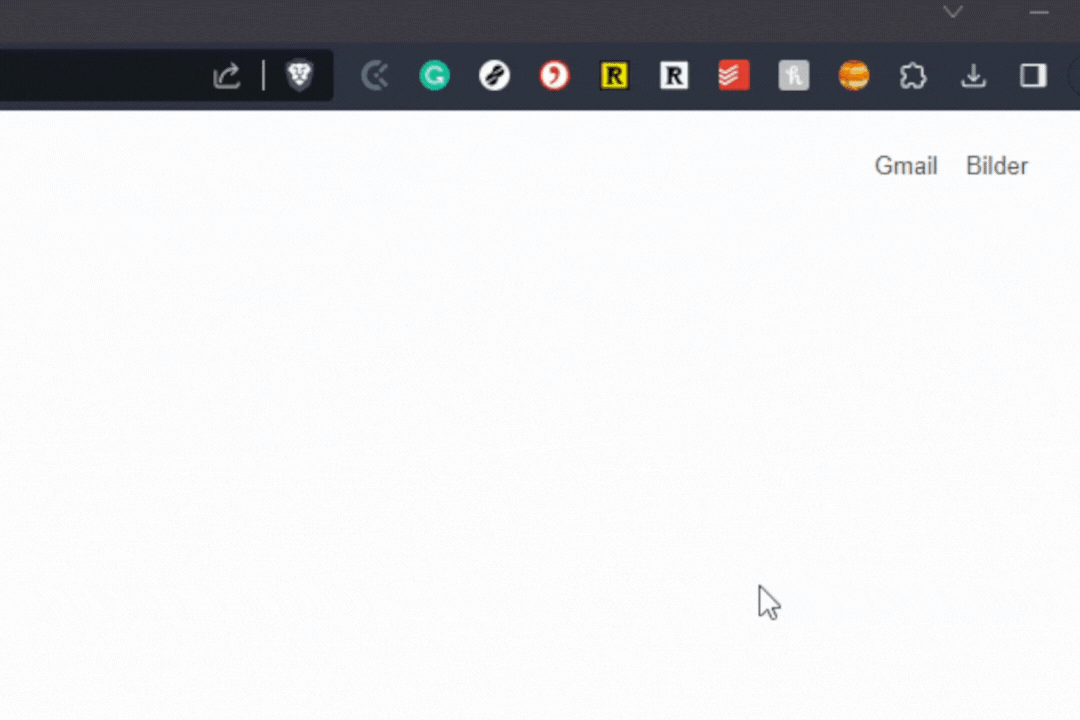
Set up a project to build a Chrome Extension
To set up the project, we will use the chrome-extension-cli. It basically sets up a basic project for us, and we only need to do some more steps before we can start building our application. Therefore we run the following commands:
- Install the CLI:
pnpm install -g chrome-extension-cli
- Set up the extension:
chrome-extension-cli calculator-extension
- Run the dev mode:
cd calculator-extension && pnpm watch
Next, we need to install the extension inside the browser. Follow these steps:
- Open
chrome://extensions
in your Browser - Enable dev mode:
- Click on “Load unpacked”
- Load the build directory as a Chrome extension.
Now you can remove the boilerplate code and start building your own extension. In the next step, we will build a calculator.
Build the calculator as a popup Chrome extension
First, we will update the public/popup.html
with the following code. It is basically only a text field and a text for the result.
Need help or want to share feedback? Join my discord community!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Calculator</title>
</head>
<body>
<div class="container">
<h1>Calculator</h1>
<form id="form">
<input id="input" type="text" placeholder="1 + 2" />
</form>
</div>
<p id="output">Result</p>
</body>
</html>
Next, we will import the styles in the head of the HTML:
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@picocss/pico@1/css/pico.min.css">
<link rel="stylesheet" href="popup.css" />
The main styles are defined by picocss and src/popup.css
will set the size of the popup window.
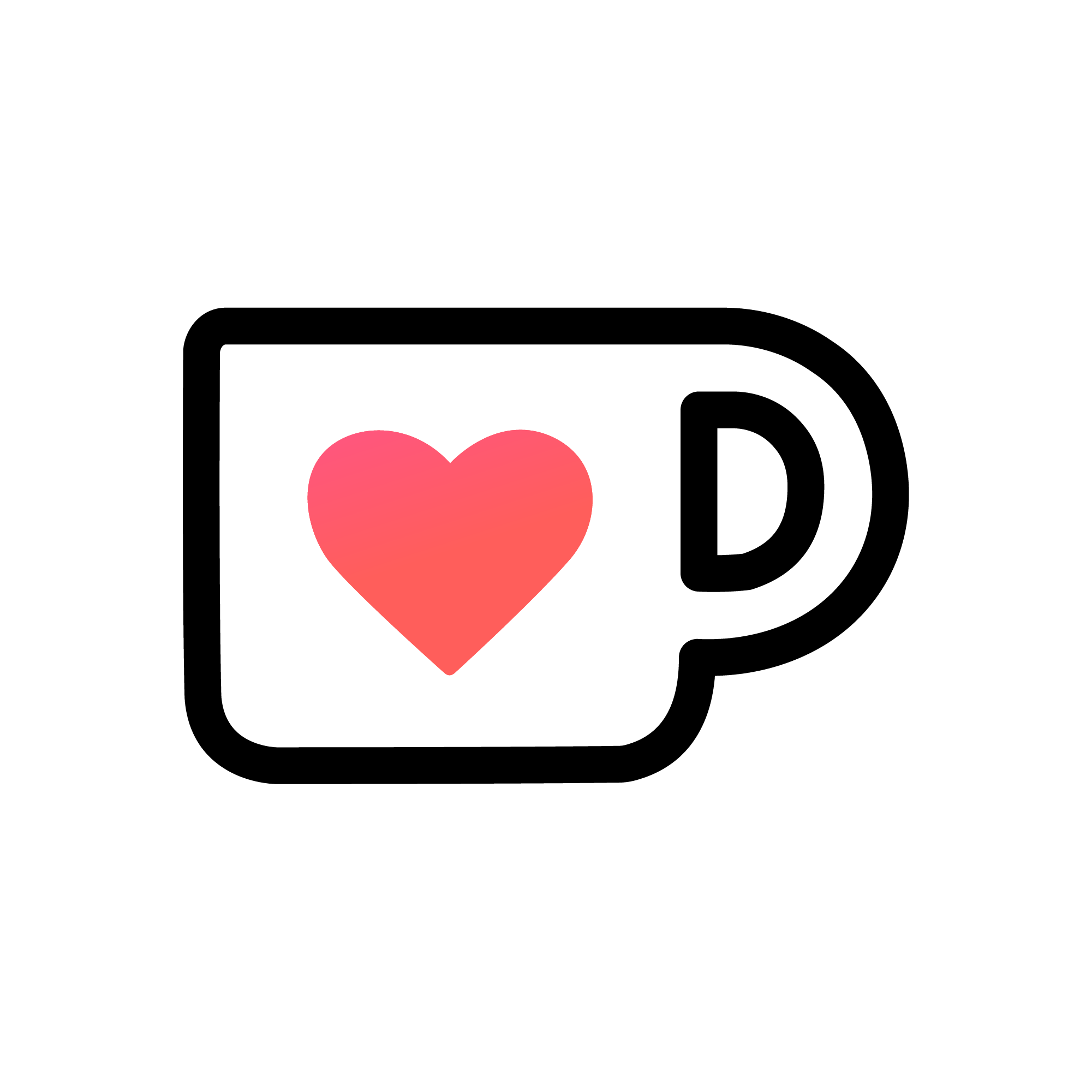
If this guide is helpful to you and you like what I do, please support me with a coffee!
body {
width: 350px;
height: 300px;
}
Lastly, we will create the calculator logic. For that, we will first install the module mathjs by running pnpm install mathjs
. Then import popup.js
at the end of the body:
<script src="popup.js"></script>
And then update the code inside with the following:
import './popup.css';
const math = require('mathjs');
const input = document.getElementById('input');
const output = document.getElementById('output');
const form = document.getElementById('form');
form.onsubmit = (e) => {
e.preventDefault();
calculate();
};
input.onclick = function () {
this.select();
};
function calculate() {
const res = math.evaluate(input.value);
output.innerHTML = res;
}
Lines 1 to 5 import mathjs and define the needed variables. Lines 7 to 10 then enable the user to press enter in the input field to start the function calculation. Next, lines 12 to 15 select the text inside the input element when it is clicked, and lastly, lines 16 to 19 define the calculate function, which runs the calculation and writes it to the result element.
With that, we have a working calculator extension, but every time we close the window, the equation and result are lost. Therefore we will use the storage API to persist both.
Use Chromes storage API to persist the equation and result
To do so, first, make sure that the “storage” permission is available in public/manifest.json
and if it is not, add it.
{
...
"permissions": [
"storage"
]
...
}
We will store both the equation and the result. Therefore we need to adjust the calculate function to the following:
function calculate() {
const res = math.evaluate(input.value);
output.innerHTML = res;
chrome.storage.local.set({ equation: input.value, result: res }).then(() => {
console.log("Value is set to " + res);
})
}
Next we need to load them whenever the window is opened. To do so, we add the following code to the end of the file:
(function (){
chrome.storage.local.get(["result","equation"]).then((result) => {
const eq = result["equation"];
const res = result["result"];
input.value = eq ? eq : '';
output.innerHTML = res ? res : 'Result';
});
})()
Conclusion
With that, you learned how to build a popup Chrome extension, with the example being a calculator app. We learned how to add scripts, CSS, and use the storage API. You can find the whole extension in GitHub here.
I hope it was helpful to you, and if you have any questions just ask!
If you liked it consider subscribing to my newsletter!
Discussion (2)
-
-
Programonaut
Glad you liked it! :)
-
Awesome