How To Manipulate Form Action Data Before sending it in SvelteKit
Do you want to manipulate the form data before sending it using a form action in SvelteKit? In this post, we will learn exactly that in a short step-by-step example!
- Example use case: Update an Item using a Form Action
- Manipulate the Form Action Data before sending it to the server in SvelteKit
- Conclusion
Example use case: Update an Item using a Form Action
Take an online donut shop that allows the owner to edit the entries using a form. Inside the database, the donut is stored with a unique id and its data. We could add the id to the form data by creating a hidden input field for the id using: <input type="hidden" name="id" value={donut.id} />
.
That is an okay approach when going for simple entries, and if there are not too many, but as soon as you have three or four and some needing calculations, it is not so cool anymore. So we want another way to add the id to the form data before the form action executed on the server receives it.
Let’s have a look at the edit donut component on the frontend (+page.svelte
):
<script lang="ts">
export let data;
</script>
<svelte:head>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@picocss/pico@1/css/pico.min.css">
</svelte:head>
<section class="container">
<h1>Edit {data.donut.name}</h1>
<form method="POST" action="?/update">
<label for="name">Name</label>
<input type="text" name="name" id="name" value="{data.donut.name}">
<label for="price">Price</label>
<input type="number" name="price" id="price" value="{data.donut.price}">
<label for="description">Description</label>
<textarea name="description" id="description">{data.donut.description}</textarea>
<button type="submit">Save</button>
</form>
</section>
And the server side form action (+page.server.ts
):
import type { Actions, PageServerLoad } from './$types';
export const actions: Actions = {
update: async ({request}) => {
const data = await request.formData();
console.log(...data);
// save the data to the database e.g. with pocketbase
// const record = await pb.collection('donuts').update(data.get('id'), data);
}
};
export const load: PageServerLoad = async ({ params }) => {
// load the data from the database e.g. with pocketbase
// const record = await pb.collection('donuts').get(params.id);
// return { donut: record };
return { donut: {
id: "vanille-donut",
name: "Vanille Donut",
description: "A donut with vanilla flavor",
price: 1,
}};
}
When we update the donut, we can see that on the backend, no id arrives.
Need help or want to share feedback? Join my discord community!
[ 'name', 'Vanille Donut' ] [ 'price', '1' ] [ 'description', 'A donut with vanilla flavor' ]
In the next step, we will change that!
Manipulate the Form Action Data before sending it to the server in SvelteKit
To manipulate the form data, we have to use the use:enhance
action (read more on it here) and run a SubmitFunction. In this function, we can access the data object before sending it to the server. So let’s do exactly that and add the id as an item in the form data:
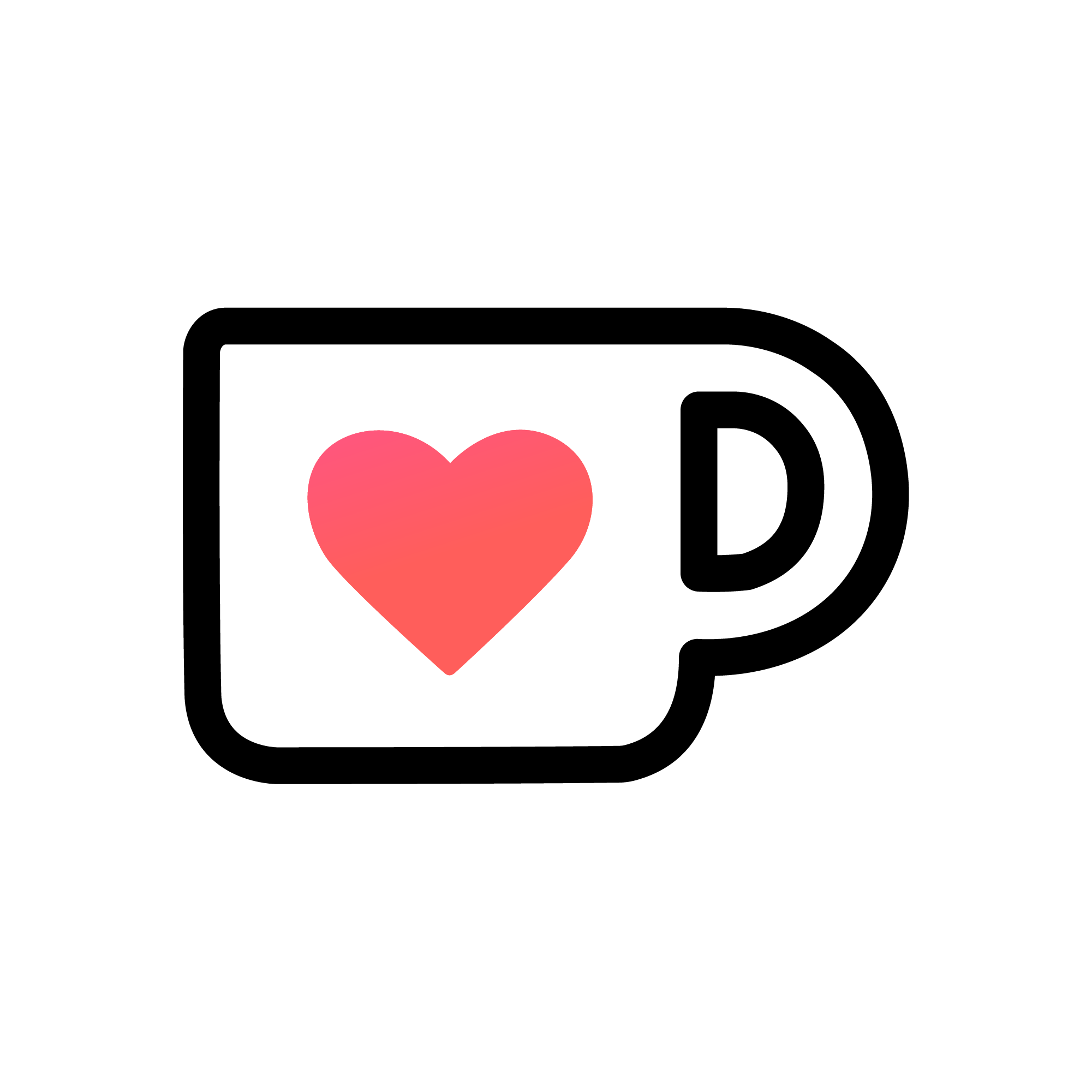
If this guide is helpful to you and you like what I do, please support me with a coffee!
<script lang="ts">
import { enhance } from '$app/forms';
export let data;
</script>
<!-- ... -->
<form method="POST" action="?/update" use:enhance={({data: formData}) => {
formData.append('id', data.donut.id);
}}>
<!-- ... -->
When we now click on the submit button again, we can see that the formData on the backend also includes the id now:
[ 'name', 'Vanille Donut' ] [ 'price', '1' ] [ 'description', 'A donut with vanilla flavor' ] [ 'id', 'vanille-donut' ]
With that, we can easily manipulate the form data before the form action sends it to the server. For the full code, check this GitHub Repository.
Conclusion
After this quick guide, we can use the full power of the SvelteKit Form Actions because we can easily manipulate the data before sending it to the server side!
In case you also want to know how to use the data that the Form Action sends back, check this guide here.
If you liked the post, consider subscribing to my newsletter, and if you have any other questions, feel free to join the discord or email me.
[convertkit form=2303042]