Pocketbase as a Framework: Records Fundamentals in Go
Using Pocketbase as a Framework enables you to easily create a one-file backend with everything you need! In this post, we will learn how to work with Pocketbase Records so that you can request, store and update data in Pocketbase’s Database. (Disclaimer: Pocketbase is still in a pretty early stage, so if you see that something in this post is not working anymore, tell me so that I can update it 😄)
- Creating Pocketbase record types in Go (coming soon)
- Requesting records stored in Pocketbase (using Go)
- Read the content of the requested Records
- Create new records (using Go)
- Update a record in Pocketbase (using Go)
- Delete a record in Pocketbase
(Find the page of the Pocketbase documentation here)
Requesting records stored in Pocketbase (using Go)
FindRecordById
// single
record, err := app.Dao().FindRecordById("posts", "RECORD_ID")
// multiple
records, err := app.Dao().FindRecordsByIds("posts", []string{"RECORD_ID1", "RECORD_ID2"})
FindFirstRecordByData (Single)
record, err := app.Dao().FindFirstRecordByData("posts", "title", "test")
FindRecordsByExpr (Multiple)
records, err := app.Dao().FindRecordsByExpr("posts",
dbx.HashExp{"category": "howto"},
)
// example using NewExp
records, err := app.Dao().FindRecordsByExpr("posts",
dbx.NewExp("LOWER(username) = {:username}", dbx.Params{"username": "John.Doe"}),
)
// example using And
records, err := app.Dao().FindRecordsByExpr("posts",
dbx.And(
dbx.HashExp{"category": "howto"},
dbx.NewExp("LOWER(username) = {:username}", dbx.Params{"username": "John.Doe"}),
)
)
Auth records
Need help or want to share feedback? Join my discord community!
// using email, username or token
user, err := app.Dao().FindAuthRecordByEmail("users", "test@example.com")
user, err := app.Dao().FindAuthRecordByUsername("users", "John.Doe")
user, err := app.Dao().FindAuthRecordByToken("JWT_TOKEN", app.Settings().RecordAuthToken.Secret)
// using id
user, err := app.Dao().FindRecordById("users", "USER_ID")
Custom query
collection, err := dao.FindCollectionByNameOrId("posts")
if err != nil {
return nil, err
}
query := dao.RecordQuery(collection).
AndWhere(dbx.HashExp{"category": "howto"}).
OrderBy("published_date DESC").
Limit(10)
rows := []dbx.NullStringMap{}
if err := query.All(&rows); err != nil {
return nil, err
}
Read the content of the requested Records
// retrieve a single record field value
record.Get("field") // -> as any
record.GetBool("field") // -> as bool
record.GetString("field") // -> as string
record.GetInt("field") // -> as int
record.GetFloat("field") // -> as float64
record.GetTime("field") // -> as time.Time
record.GetDateTime("field") // -> as types.DateTime
record.GetStringSlice("field") // -> as []string
// unmarshal a single json field value into the provided result
record.UnmarshalJSONField("field", &result)
Create new records (using Go)
With data validation
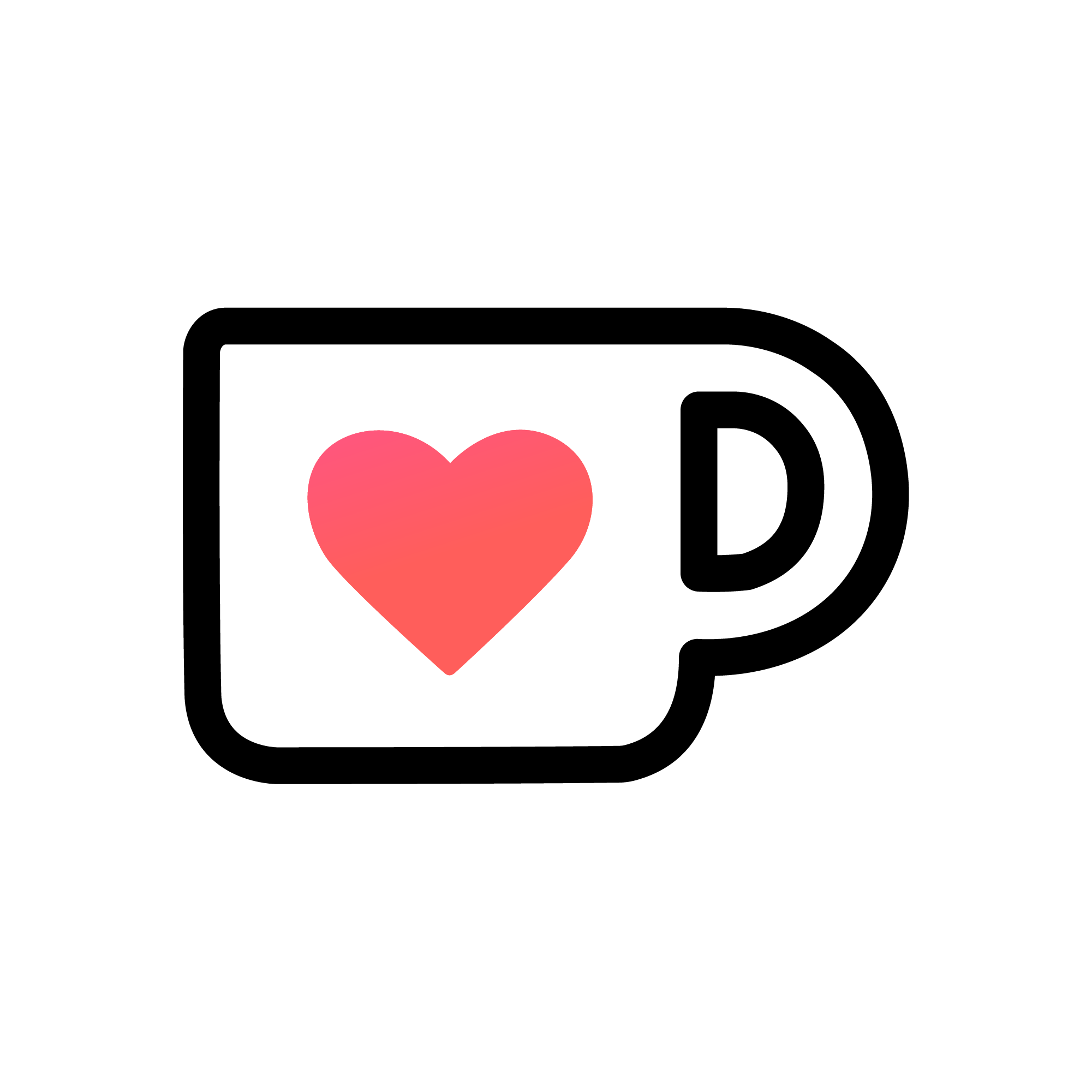
If this guide is helpful to you and you like what I do, please support me with a coffee!
// get the collection
collection, err := app.Dao().FindCollectionByNameOrId("scheduled")
if err != nil {
return err
}
// create a new post record from the collection
postRecord := models.NewRecord(collection)
form := forms.NewRecordUpsert(app, postRecord)
form.LoadData(map[string]any{
"title": "Example",
"excerpt": "Short excerpt",
//...
})
if err := form.Submit(); err != nil {
return err
}
A cool thing is that you can continue using the new postRecord and update it again:
form := forms.NewRecordUpsert(app, postsRecord)
form.LoadData(map[string]any{
"title": "Update",
})
if err := form.Submit(); err != nil {
return err
}
Without data validation
collection, err := app.Dao().FindCollectionByNameOrId("posts")
if err != nil {
return err
}
postRecord := models.NewRecord(collection)
postRecord.Set("title", "Example")
postRecord.Set("excerpt", "Short excerpt")
//...
if err := app.Dao().SaveRecord(postRecord); err != nil {
return err
}
Update a record in Pocketbase (using Go)
With data validation
// get the record
postRecord, err := app.Dao().FindRecordById("posts", "RECORD_ID")
if err != nil {
return err
}
form := forms.NewRecordUpsert(app, postRecord)
form.LoadData(map[string]any{
"title": "Example",
"excerpt": "Short excerpt",
//...
})
if err := form.Submit(); err != nil {
return err
}
Without data validation
postRecord, err := app.Dao().FindRecordById("posts", "RECORD_ID")
if err != nil {
return err
}
postRecord.Set("title", "Example")
postRecord.Set("excerpt", "Short excerpt")
if err := app.Dao().SaveRecord(postRecord); err != nil {
return err
}
Delete a record in Pocketbase
postRecord, err := app.Dao().FindRecordById("posts", "RECORD_ID")
if err != nil {
return err
}
if err := app.Dao().DeleteRecord(postRecord); err != nil {
return err
}
Conclusion
Pocketbase is a great framework for creating a one-file backend with everything you need. In this post, you learned how to work with Pocketbase Records to request, store, and update data in Pocketbase’s Database.
In case you have any questions regarding this post, send me an email at mail@programonaut.com.
And if you want to learn more about Pocketbase and why I prefer it over something like Supabase, you can check this post here, or if you want to learn how to create a simple chat application with a login screen, check these two posts here and here.
In case you found this post helpful, consider subscribing to my monthly newsletter to be updated on all my new posts 🙂
[convertkit form=2303042]